These keys must be generated individually, follow the steps below to generate them without error.
Generate auth_code with sc_api_gc_get_url macro
The sc_api_gc_get_url macro generates a URL for google account user authentication used for API configuration.
It has two parameters:
- app_name - Receive the app_name, available in your api configuration. This parameter accepts strings or variable.
- json_oauth - Receive the content of the json file, this file is available for download in your api settings. This parameter accepts strings or variable.
Follow the steps bellow.
1. Create a blank application in your project, with the objective of obtaining the auth_code.
2. In the onExecute event, add the code below.
The values assigned to the variables $app_name and $json_oauth, in the example below, are for illustration only and should be changed by your account data.
Code example
- // $app_name should receive the app name configured in your google account
- $app_name = 'scriptcase';
- // $json_oauth should receive the contents of the json file available for download in your google app's settings.
- $json_oauth = '{"installed":{"client_id":"238650139811-eqrje3lqhj9a404iuduh9l5kvk5i13ng.apps.googleusercontent.com","project_id":"sample-294791","auth_uri":"https://accounts.google.com/o/oauth2/auth","token_uri":"https://oauth2.googleapis.com/token","auth_provider_x519_cert_url":"https://www.googleapis.com/oauth2/v1/certs","client_secret":"E-Z_t8PU-8u0BFwumHRorbTl","redirect_uris":["urn:ietf:wg:oauth:2.0:oob","http://localhost"]}}';
- // Generates the account authentication link
- $url = sc_api_gc_get_url($app_name, $json_oauth);
- // Redirects the app to the link generated by the macro
- sc_redir($url);
Google authentication
3. Then run the application.
You will be asked to login with the account used to configure the API.
4. After login, access permission will be requested:
4. Then the code will be generated.
Store the code in a txt file, it will be needed for us to generate the token_code.
Possible errors
This error occurs when the value informed in the json_oauth parameter is wrong.
Open the json file again, copy the entire contents of the file, it must be informed in full.
Generate token_code with sc_api_gc_get_obj macro
The sc_api_gc_get_obj macro generates the token_code.
It has four parameters:
- app_name - Receive the app_name, available in your api configuration. This parameter accepts strings or variable.
- json_oauth - Receives the content of the json file, this file is available for download in your API settings. This parameter accepts strings or variable.
- auth_code - Receives the auth_code generated in the previous step or another valid auth_code that you have already generated.
Follow the steps below.

This walkthrough should only be performed for each auth_code generated. If any problem occurs when generating the token_code, it will be necessary to generate a new auth_code.
In the same blank application, created to generate the auth_code, it is also possible to generate the token_code, for that follow the steps below.
5. Comment on the lines highlighted below:
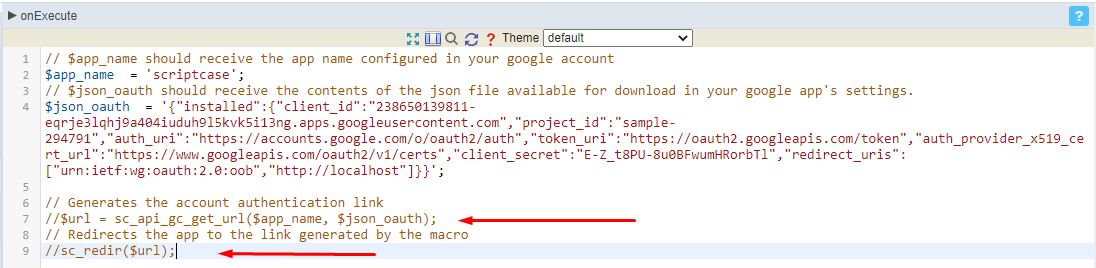
6. After the sc_redir line, which should be commented out, add the lines below.
Code example
- // Receives auth_code generated in the previous step
- $auth_code = '4/1AY0e-g4BjstStsxAJd7A5jjlzBifl5AWSS9x04xhwp6Cyh2JON0i3r2oNd4';
- $auth_token = sc_api_gc_get_obj($app_name, $json_oauth, $auth_code);
- echo $auth_token;
The code should be looking like this at the moment.
7. Then run the application. The token_code value must be displayed on the screen.
Store the token in a .txt file.
Example of generated token_code:
- {"access_token":"ya29.a1AfH6SMBSExys1mg0_4aaZ1H8iVNUtZ-2iid4zIjvywOpPDpL5_fhpuYu_Evgqj14Zll1mu0DKeX-UPBBbkIY33T_hIwUKNi6h5GVmE8RSt7vwj1N8rmM_ae3EjOBMDIaS_F2vkZ8ZzpnBA0V38WwYBxBNR1C","expires_in":3599,"refresh_token":"1\/\/0heh4c5k0p_OqCgYIARAAGBESNwF-L2IrTOLtSoBOr-qdK4gDtSkyXtLYh99S1DGG6Y0x6sBwNu2sVnlqEFczG7-zEIBvCd8Bb6Q","scope":"https:\/\/www.googleapis.com\/auth\/drive","token_type":"Bearer","created":1623154166}

The sc_api_gc_get_obj macro must be run only once for each auth_code generated.
After getting the token_code, the auth_token parameter must be changed so that a new token_code is obtained. Otherwise, Fatal error: Uncaught InvalidArgumentException: Invalid token format, described below, will be displayed when running the application.
Possible errors when generating token_code.
This error occurs when the sc_api_gc_get_obj macro is run a second time without the auth_code values having been changed.
In this case, it will be necessary to generate a new auth_code, to get a new one as seen at the beginning of the article. Return to step Generate auth_code with sc_api_gc_get_url macro